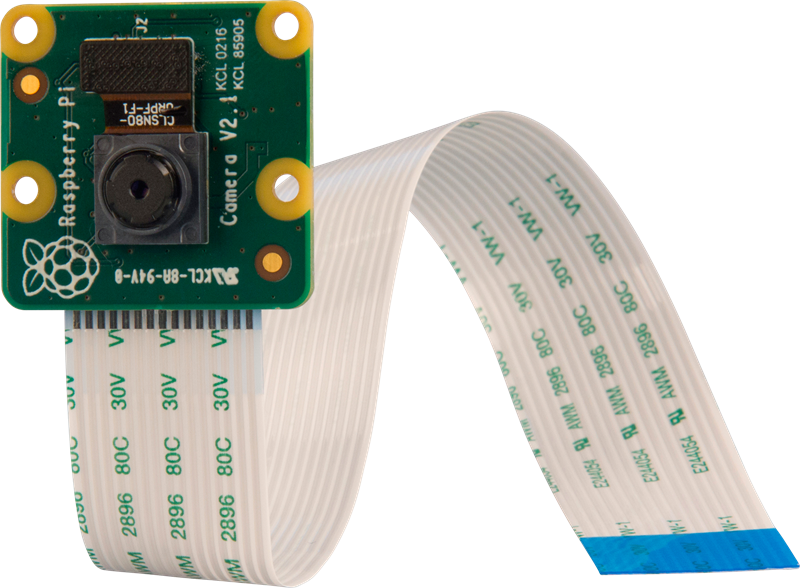
PiCamの高速化
2017年7月20日 - 未分類
RaspberryPiのpicameraは、小さくて優秀ですが、読み込み速度があまり早くないなって思っていました。ふと、Therad化すれば、早くなるんじゃないかって考えて調べるとありました。
Therad化することで、カメラ画像の読込が3,4倍早くなります。使い方も簡単です。ほぼ、ストレスなく動くように見えます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 | # import the necessary packages from picamera.array import PiRGBArray from picamera import PiCamera from threading import Thread import cv2 import time class PiVideoStream: def __init__( self , resolution = ( 320 , 240 ), framerate = 32 ): # initialize the camera and stream self .camera = PiCamera() self .camera.resolution = resolution self .camera.framerate = framerate self .rawCapture = PiRGBArray( self .camera, size = resolution) self .stream = self .camera.capture_continuous( self .rawCapture, format = "bgr" , use_video_port = True ) self .stopped = False # initialize the frame and the variable used to indicate # if the thread should be stopped self .frame = None self .stopped = False #self.camera.resolution = (640, 480) #self.camera.framerate = 32 self .camera.sharpness = 0 self .camera.contrast = 0 self .camera.brightness = 50 self .camera.saturation = 0 self .camera.ISO = 0 self .camera.video_stabilization = False self .camera.exposure_compensation = 0 #self.camera.exposure_mode = 'off' self .camera.awb_mode = 'flash' self .camera.meter_mode = 'average' self .camera.image_effect = 'none' self .camera.color_effects = None self .camera.rotation = 0 self .camera.hflip = True self .camera.vflip = True self .camera.crop = ( 0.0 , 0.0 , 1.0 , 1.0 ) def start( self ): # start the thread to read frames from the video stream Thread(target = self .update, args = ()).start() return self def update( self ): # keep looping infinitely until the thread is stopped for f in self .stream: # grab the frame from the stream and clear the stream in # preparation for the next frame self .frame = f.array self .rawCapture.truncate( 0 ) # if the thread indicator variable is set, stop the thread # and resource camera resources if self .stopped: self .stream.close() self .rawCapture.close() self .camera.close() return def read( self ): # return the frame most recently read return self .frame def stop( self ): # indicate that the thread should be stopped self .stopped = True def seek( self ): self .rawCapture.seek( - 1 , 2 ) vs = PiVideoStream(( 640 , 480 ), 32 ).start() time.sleep( 2.0 ) def PiCamCapture(): return vs.read() def PiCamCaptureSeek(): return vs.seek() def PiCamCaptureStop(): vs.stop() if __name__ = = '__main__' : # cv2.namedWindow( 'Frame' , cv2.WINDOW_NORMAL) while True : frame = PiCamCapture() # check to see if the frame should be displayed to our screen cv2.imshow( "Frame" , frame) key = cv2.waitKey( 1 ) & 0xFF if key = = 27 : break # do a bit of cleanup cv2.destroyAllWindows() PiCamCaptureStop() |